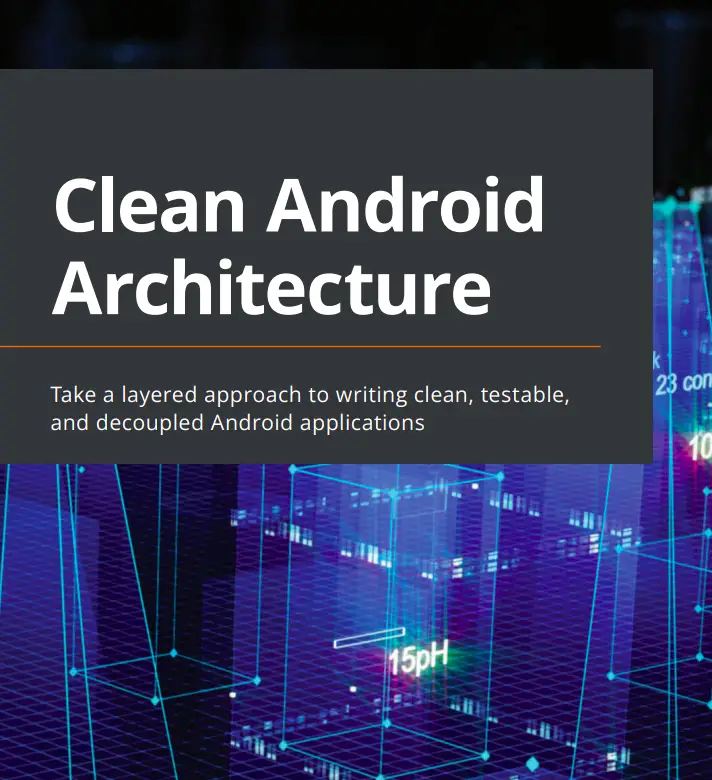
What this book covers
Chapter 1 : Getting Started with Clean Architecture, starts by presenting the evolution of Android apps with regards to how business logic was structured, and the problems caused by these approaches. It will then transition to how certain patterns were applied to tackle these issues, revealing other sets of issues. Finally, the concept of clean architecture will be introduce, as well as how its principles can be used to solve some of the problems presented previously
Chapter 2 : Deep Diving into Data Sources, covers what Android tools and frameworks are available to use with regard to the implementation of the data layer and details and expands on the ones that will be used later in the book, such as Kotlin flows and coroutines, Retrofit, Room, and DataStore.
Chapter 3 : Understanding Data Presentation on Android, covers what Android tools and frameworks are available to use with regard to the implementation of the presentation layer and will detail and expand on the ones that will be used later in the book, such as Android ViewModel and Jetpack Compose.
Chapter 4 : Managing Dependencies in Android Applications, provides a quick overview of dependency injection and how it works. It briefly explores some of the dependency injection tools available for Android development, ending with the Hilt dependency injection framework, about which it goes into a more detailed explanation because it will be used in many of the exercises in the book
Chapter 5 : Building the Domain of an Android Application, describes how to build a domain layer and what components are part of this layer. You will learn about entities and use cases or interactors and what roles they play when it comes to designing the architecture of your application.
Chapter 6 : Assembling a Repository, covers the Data layer and the responsibilities this layer has when it comes to managing an application’s data, and how it can use the Repository pattern to achieve this.
Chapter 7 : Building Data Sources, continues the exploration into the Data layer and some examples of data sources that can be defined in Android. You will learn about using remote data sources to load data from various servers as well as local data sources such as Room and DataStore.
Chapter 8 : Implementing an MVVM Architecture, presents the MVVM architecture pattern and how it can be used in an application’s presentation layer. You will learn how to use the Android ViewModel and LiveData to build an app with MVVM and integrate use cases into the ViewModel.
Chapter 9 : Implementing an MVI Architecture, presents the MVI architecture pattern and how it can be used in an application’s presentation layer. You will learn how to use Kotlin flows and Android ViewModel to implement the MVI pattern.
Chapter 10 : Putting It All Together, covers the benefits of clean architecture by analyzing an example of an application that implements the concepts and then adding instrumentation tests with Espresso and Jetpack Compose. The introduction of UI tests serves as a good example of how we can inject and change certain behaviors in the application for testing purposes without needing to modify the application’s code.
Leave a Reply