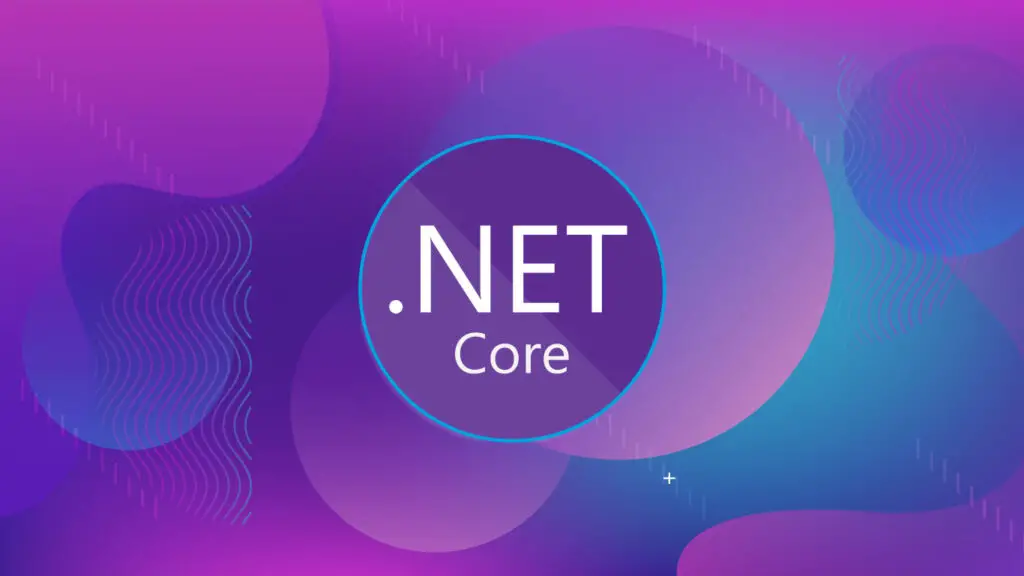
Best Dot NET Core interview questions and answers
1. What is .NET Core?
Answer:
The .NET Core platform is a new .NET stack that is optimized for open source development and agile delivery on NuGet.
.NET Core has two major components. It includes a small runtime that is built from the same codebase as the .NET Framework CLR. The .NET Core runtime includes the same GC and JIT (RyuJIT), but doesn’t include features like Application Domains or Code Access Security. The runtime is delivered via NuGet, as part of the ASP.NET Core package.
.NET Core also includes the base class libraries. These libraries are largely the same code as the .NET Framework class libraries, but have been factored (removal of dependencies) to enable to ship a smaller set of libraries. These libraries are shipped as System.*
NuGet packages on NuGet.org.
Source: https://stackoverflow.com/questions/26908049/what-is-net-core
2. What is MSIL?
Answer:
When we compile our .NET code then it is not directly converted to native/binary code; it is first converted into intermediate code known as MSIL code which is then interpreted by the CLR. MSIL is independent of hardware and the operating system. Cross language relationships are possible since MSIL is the same for all .NET languages. MSIL is further converted into native code.
Source: https://www.c-sharpcorner.com/UploadFile/8ef97c/interview-question-on-net-framework-or-clr/
3. What is .NET Standard and why we need to consider it?
Answer:
- .NET Standard solves the code sharing problem for .NET developers across all platforms by bringing all the APIs that you expect and love across the environments that you need: desktop applications, mobile apps & games, and cloud services:
- .NET Standard is a set of APIs that all .NET platforms have to implement. This unifies the .NET platforms and prevents future fragmentation.
- .NET Standard 2.0 will be implemented by .NET Framework, .NET Core, and Xamarin. For .NET Core, this will add many of the existing APIs that have been requested.
- .NET Standard 2.0 includes a compatibility shim for .NET Framework binaries, significantly increasing the set of libraries that you can reference from your .NET Standard libraries.
- .NET Standard will replace Portable Class Libraries (PCLs) as the tooling story for building multi-platform .NET libraries.
Source: https://stackoverflow.com/questions/42939454/what-is-the-difference-between-net-core-and-net-standard-class-library-project
4. What is CTS?
Answer:
The Common Type System (CTS) standardizes the data types of all programming languages using .NET under the umbrella of .NET to a common data type for easy and smooth communication among these .NET languages.
CTS is designed as a singly rooted object hierarchy with System.Object
as the base type from which all other types are derived. CTS supports two different kinds of types:
- Value Types: Contain the values that need to be stored directly on the stack or allocated inline in a structure. They can be built-in (standard primitive types), user-defined (defined in source code) or enumerations (sets of enumerated values that are represented by labels but stored as a numeric type).
- Reference Types: Store a reference to the value‘s memory address and are allocated on the heap. Reference types can be any of the pointer types, interface types or self-describing types (arrays and class types such as user-defined classes, boxed value types and delegates).
Source: https://www.c-sharpcorner.com/UploadFile/8ef97c/interview-question-on-net-framework-or-clr/
5. What is a .NET application domain?
Answer:
It is an isolation layer provided by the .NET runtime. As such, App domains live with in a process (1 process can have many app domains) and have their own virtual address space.
App domains are useful because:
- They are less expensive than full processes
- They are multithreaded
- You can stop one without killing everything in the process
- Segregation of resources/config/etc
- Each app domain runs on its own security level
Source: https://stackoverflow.com/questions/1094478/what-is-a-net-application-domain
6. What is an unmanaged resource?
Answer:
Use that rule of thumb:
- If you found it in the Microsoft .NET Framework: it’s managed.
- If you went poking around MSDN yourself, it’s unmanaged.
Anything you’ve used P/Invoke calls to get outside of the nice comfy world of everything available to you in the .NET Framwork is unmanaged – and you’re now responsible for cleaning it up.
Source: https://stackoverflow.com/questions/538060/proper-use-of-the-idisposable-interface
7. What is CLR?
Answer:
The CLR stands for Common Language Runtime and it is an Execution Environment. It works as a layer between Operating Systems and the applications written in .NET languages that conforms to the Common Language Specification (CLS). The main function of Common Language Runtime (CLR) is to convert the Managed Code into native code and then execute the program.
Source: https://www.c-sharpcorner.com/UploadFile/8ef97c/interview-question-on-net-framework-or-clr/
8. What is the difference between String and string in C#?
Answer:
string
is an alias in C# for System.String
. So technically, there is no difference. It’s like int
vs. System.Int32
.
As far as guidelines, it’s generally recommended to use string
any time you’re referring to an object.
string place = "world";
Likewise, it’s generally recommended to use String
if you need to refer specifically to the class.
string greet = String.Format("Hello {0}!", place);
Source: https://stackoverflow.com/questions/618535/difference-between-decimal-float-and-double-in-net
9. What is .NET Standard?
Answer:
The .NET Standard is a formal specification of .NET APIs that are intended to be available on all .NET implementations.
Source: https://docs.microsoft.com/en-us/dotnet/standard/net-standard
10. What is the .NET Framework?
Answer:
The .NET is a Framework, which is a collection of classes of reusable libraries given by Microsoft to be used in other .NET applications and to develop, build and deploy many types of applications on the Windows platform including the following:
- Console Applications
- Windows Forms Applications
- Windows Presentation Foundation (WPF) Applications
- Web Applications
- Web Services
- Windows Services
- Services-oriented applications using Windows Communications Foundation (WCF)
- Workflow-enabled applications using Windows Workflow Foundation(WF)
Source: https://www.c-sharpcorner.com/UploadFile/8ef97c/interview-question-on-net-framework-or-clr/
12. What are some characteristics of .NET Core?
Answer:
- Flexible deployment: Can be included in your app or installed side-by-side user- or machine-wide.
- Cross-platform: Runs on Windows, macOS and Linux; can be ported to other OSes. The supported Operating Systems (OS), CPUs and application scenarios will grow over time, provided by Microsoft, other companies, and individuals.
- Command-line tools: All product scenarios can be exercised at the command-line.
- Compatible: .NET Core is compatible with .NET Framework, Xamarin and Mono, via the .NET Standard Library.
- Open source: The .NET Core platform is open source, using MIT and Apache 2 licenses. Documentation is licensed under CC-BY. .NET Core is a .NET Foundation project.
- Supported by Microsoft: .NET Core is supported by Microsoft, per .NET Core Support
Source: https://stackoverflow.com/questions/26908049/what-is-net-core
13. What is the difference between decimal, float and double in .NET?
Answer:
Problem
When would someone use one of these?
Precision is the main difference.
- Float – 7 digits (32 bit)
- Double-15-16 digits (64 bit)
- Decimal -28-29 significant digits (128 bit)
As for what to use when:
- For values which are “naturally exact decimals” it’s good to use decimal. This is usually suitable for any concepts invented by humans: financial values are the most obvious example, but there are others too. Consider the score given to divers or ice skaters, for example.
- For values which are more artefacts of nature which can’t really be measured exactly anyway, float/double are more appropriate. For example, scientific data would usually be represented in this form. Here, the original values won’t be “decimally accurate” to start with, so it’s not important for the expected results to maintain the “decimal accuracy”. Floating binary point types are much faster to work with than decimals.
Source: https://stackoverflow.com/questions/618535/difference-between-decimal-float-and-double-in-net
14. What’s the difference between SDK and Runtime in .NET Core?
Answer:
- The SDK is all of the stuff that is needed/makes developing a .NET Core application easier, such as the CLI and a compiler.
- The runtime is the “virtual machine” that hosts/runs the application and abstracts all the interaction with the base operating system.
Source: https://stackoverflow.com/questions/47733014/whats-the-difference-between-sdk-and-runtime-in-net-core
15. Name some CLR services?
Answer:
CLR services
- Assembly Resolver
- Assembly Loader
- Type Checker
- COM marshalled
- Debug Manager
- Thread Support
- IL to Native compiler
- Exception Manager
- Garbage Collector
Source: https://www.c-sharpcorner.com/UploadFile/8ef97c/interview-question-on-net-framework-or-clr/
16. Explain two types of deployment for .NET Core applications
Answer:
- Framework-dependent deployment (FDD) – it relies on the presence of a shared system-wide version of .NET Core on the target system. The app contains only its own code and any third-party dependencies that are outside of the .NET Core libraries. FDDs contain .dll files that can be launched by using the dotnet utility from the command line.
dotnet app.dll
* **Self-contained deployment** - Unlike FDD, a self-contained deployment (SCD) doesn't rely on the presence of shared components on the target system. All components, including both the .NET Core libraries and the .NET Core runtime, are included with the application and are isolated from other .NET Core applications. SCDs include an executable (such as app.exe on Windows platforms for an application named app), which is a renamed version of the platform-specific .NET Core host, and a .dll file (such as app.dll), which is the actual application.
Source: https://docs.microsoft.com/en-us/dotnet/core/deploying/index
17. Explain what is included in .NET Core?
Answer:
- A .NET runtime, which provides a type system, assembly loading, a garbage collector, native interop and other basic services.
- A set of framework libraries, which provide primitive data types, app composition types and fundamental utilities.
- A set of SDK tools and language compilers that enable the base developer experience, available in the .NET Core SDK.
- The ‘dotnet’ app host, which is used to launch .NET Core apps. It selects the runtime and hosts the runtime, provides an assembly loading policy and launches the app. The same host is also used to launch SDK tools in much the same way.
Source: https://stackoverflow.com/questions/26908049/what-is-net-core
18. Is there a way to catch multiple exceptions at once and without code duplication?
Answer:
Problem
Consider: try { WebId = new Guid(queryString[“web”]); } catch (FormatException) { WebId = Guid.Empty; } catch (OverflowException) { WebId = Guid.Empty; }
Is there a way to catch both exceptions and only call the WebId = Guid.Empty
call once?
Catch System.Exception and switch on the types:
catch (Exception ex)
{
if (ex is FormatException || ex is OverflowException)
{
WebId = Guid.Empty;
return;
}
<span class="token cVar">throw</span><span class="token cBase">;</span>
}
Source: https://stackoverflow.com/questions/125319/should-using-directives-be-inside-or-outside-the-namespace
19. What is Kestrel?
Answer:
- Kestrel is a cross-platform web server built for ASP.NET Core based on libuv – a cross-platform asynchronous I/O library.
- It is a default web server pick since it is used in all ASP.NET Core templates.
- It is really fast.
- It is secure and good enough to use it without a reverse proxy server. However, it is still recommended that you use IIS, Nginx or Apache or something else.
Source: http://www.talkingdotnet.com/asp-net-core-interview-questions/
20. What is difference between .NET Core and .NET Framework?
Answer:
.NET as whole now has 2 flavors:
- .NET Framework
- .NET Core
.NET Core and the .NET Framework have (for the most part) a subset-superset relationship. .NET Core is named “Core” since it contains the core features from the .NET Framework, for both the runtime and framework libraries. For example, .NET Core and the .NET Framework share the GC, the JIT and types such as String
and List
.
.NET Core was created so that .NET could be open source, cross platform and be used in more resource-constrained environments.
Source: https://stackoverflow.com/questions/10448516/apache-jmeter-listener-results-interpretation
21. What’s the difference between .NET Core, .NET Framework, and Xamarin?
Answer:
- .NET Framework is the “full” or “traditional” flavor of .NET that’s distributed with Windows. Use this when you are building a desktop Windows or UWP app, or working with older ASP.NET 4.6+.
- .NET Core is cross-platform .NET that runs on Windows, Mac, and Linux. Use this when you want to build console or web apps that can run on any platform, including inside Docker containers. This does not include UWP/desktop apps currently.
- Xamarin is used for building mobile apps that can run on iOS, Android, or Windows Phone devices.
Xamarin usually runs on top of Mono, which is a version of .NET that was built for cross-platform support before Microsoft decided to officially go cross-platform with .NET Core. Like Xamarin, the Unity platform also runs on top of Mono.
Source: https://stackoverflow.com/questions/38063837/whats-the-difference-between-net-core-net-framework-and-xamarin
22. What is .NET Standard?
Answer:
- .NET Standard solves the code sharing problem for .NET developers across all platforms by bringing all the APIs that you expect and love across the environments that you need: desktop applications, mobile apps & games, and cloud services
- .NET Standard is a set of APIs that all .NET platforms have to implement. This unifies the .NET platforms and prevents future fragmentation.
- .NET Standard 2.0 will be implemented by .NET Framework, .NET Core, and Xamarin. For .NET Core, this will add many of the existing APIs that have been requested.
- .NET Standard 2.0 includes a compatibility shim for .NET Framework binaries, significantly increasing the set of libraries that you can reference from your .NET Standard libraries.
- .NET Standard will replace Portable Class Libraries (PCLs) as the tooling story for building multi-platform .NET libraries.
Source: http://www.talkingdotnet.com/asp-net-core-interview-questions/
23. What about NuGet packages and packages.config?
Answer:
There is no more packages.config file. All packages are now managed within .csproj file.
The .csproj file has been cleaned up and it also serves the role of packages.config(or package.json for Node devs). That means that’s where your packages and versions will be saved.
NuGet packages are the unit of reference and they can depend on other NuGet packages, but also they can depend on projects. And as before, projects can also depend on NuGet packages and other projects. That means that projects and NuGet packages are interchangeable.
With .NET Core, you can easily turn your projects into NuGet packages, with one click inside of the properties.
Source: https://codingblast.com/asp-net-core-interview-questions/
24. Talk about new .csproj file?
Answer:
.csproj file is now used as a place where we manage the NuGet packages for your application.
File explorer and project explorer are now in sync. For .NET Core projects, you can easily drop a file from file explorer into a project or delete it from the file system and it will be gone from the project. No more source files in a .csproj file.
You can now edit the .csproj file directly without unloading the project.
Source: https://codingblast.com/asp-net-core-interview-questions/
25. Why to use of the IDisposable interface?
Answer:
The “primary” use of the IDisposable
interface is to clean up unmanaged resources. Note the purpose of the Dispose pattern is to provide a mechanism to clean up both managed and unmanaged resources and when that occurs depends on how the Dispose method is being called.
Source: https://stackoverflow.com/questions/538060/proper-use-of-the-idisposable-interface
26. What does Common Language Specification (CLS) mean?
Answer:
The Common Language Specification (CLS) is a fundamental set of language features supported by the Common Language Runtime (CLR) of the .NET Framework. CLS is a part of the specifications of the .NET Framework. CLS was designed to support language constructs commonly used by developers and to produce verifiable code, which allows all CLS-compliant languages to ensure the type safety of code. CLS includes features common to many object-oriented programming languages. It forms a subset of the functionality of common type system (CTS) and has more rules than defined in CTS.
Source: https://www.techopedia.com/definition/25318/common-language-specification-cls-net
27. Explain the difference between Task and Thread in .NET
Answer:
- Thread represents an actual OS-level thread, with its own stack and kernel resources. Thread allows the highest degree of control; you can Abort() or Suspend() or Resume() a thread, you can observe its state, and you can set thread-level properties like the stack size, apartment state, or culture. ThreadPool is a wrapper around a pool of threads maintained by the CLR.
- The Task class from the Task Parallel Library offers the best of both worlds. Like the ThreadPool, a task does not create its own OS thread. Instead, tasks are executed by a TaskScheduler; the default scheduler simply runs on the ThreadPool. Unlike the ThreadPool, Task also allows you to find out when it finishes, and (via the generic Task) to return a result.
Source: https://stackoverflow.com/questions/13429129/task-vs-thread-differences
28. Explain the difference between “managed” and “unmanaged” code?
Answer:
- Managed code is not compiled to machine code but to an intermediate language which is interpreted and executed by some service on a machine and is therefore operating within a (hopefully!) secure framework which handles dangerous things like memory and threads for you. It runs on the CLR (Common Language Runtime), which, among other things, offers services like garbage collection, run-time type checking, and reference checking. So, think of it as, “My code is managed by the CLR.”
- Unmanaged code is compiled to machine code and therefore executed by the OS directly. It therefore has the ability to do damaging/powerful things Managed code does not. This is how everything used to work, so typically it’s associated with old stuff like .dlls
Source: https://stackoverflow.com/questions/3563870/difference-between-managed-and-unmanaged
29. What is CoreCLR?
Answer:
CoreCLR is the .NET execution engine in .NET Core, performing functions such as garbage collection and compilation to machine code.
Consider:
Source: https://blogs.msdn.microsoft.com/dotnet/2015/02/03/coreclr-is-now-open-source/
30. What is JIT compiler?
Answer:
Before a computer can execute the source code, special programs called compilers must rewrite it into machine instructions, also known as object code. This process (commonly referred to simply as “compilation”) can be done explicitly or implicitly.
Implicit compilation is a two-step process:
- The first step is converting the source code to intermediate language (IL) by a language-specific compiler.
- The second step is converting the IL to machine instructions. The main difference with the explicit compilers is that only executed fragments of IL code are compiled into machine instructions, at runtime. The .NET framework calls this compiler the JIT (Just-In-Time) compiler.
Source: https://www.telerik.com/blogs/understanding-net-just-in-time-compilation
Leave a Reply