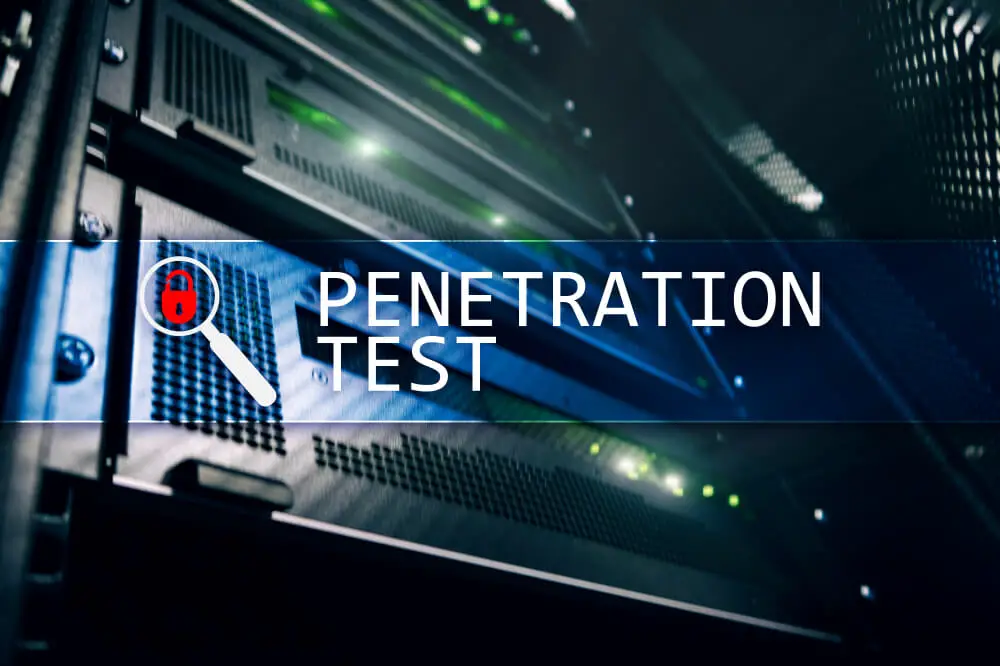
Saker is a flexible Penetration testing auxiliary suite.
Table of contents
Features
brief introduction for support features:
- scan website
- infomation gathering
- framework fingerprint
- fuzz web request
- XSS
- SQL injection
- SSRF
- XXE
- …
- subdomain gathering
- port scanner
- bruteforce
- web dir
- zip password
- domain
- …
- auxiliary servers
- dns rebinding
- ssrf
- xss
- third party api integration
- censys
- crtsh
- fofa
- github
- shodan
- sqlmap
- threadcrowd
- …
Quick Setup
latest version
pip install -U git+https://github.com/lylemi/saker
git clone https://github.com/LyleMi/Saker.git
pip install -r requirements.txt
python setup.py install
stable version
pip install Saker
develop install
add /path/to/saker to PYTHONPATH
export PYTHONPATH=/path/to/saker:$PYTHONPATH
Example Cases
Scan Website
from saker.core.scaner import Scanner
s = Scanner("http://127.0.0.1")
s.scan(filename="index.php", ext="php")
or by shell
python -m saker scan usage: main.py [options] Saker Scanner optional arguments: -h, --help show this help message and exit -s, --scan run with list model -f file, --file file scan specific file -e ext, --ext ext scan specific ext -i, --info get site info -u URL, --url URL define specific url -p PROXY, --proxy PROXY proxy url -t INTERVAL, --timeinterval INTERVAL scan time interval, random sleep by default
Fuzz Website
from saker.core.mutator import Mutator options = { "url": "http://127.0.0.1:7777/", "params": { "test": "test" } } m = Mutator(options) m.fuzz('url') m.fuzz('params', 'test')
or by shell
python -m saker fuzz usage: [options] Saker Fuzzer optional arguments: -h, --help show this help message and exit -u URL, --url URL define specific url -m METHOD, --method METHOD request method, use get as default -p PARAMS, --params PARAMS request params, use empty string as default -d DATA, --data DATA request data, use empty string as default -H HEADERS, --headers HEADERS request headers, use empty string as default -c COOKIES, --cookies COOKIES request cookies, use empty string as default -P PART, --part PART fuzz part, could be url / params / data / ... -k KEY, --key KEY key to be fuzzed -v VULN, --vuln VULN Vulnarability type to be fuzzed -t INTERVAL, --timeinterval INTERVAL scan time interval, random sleep by default
Port Scanner
python -m saker port usage: [options] Saker Port Scanner optional arguments: -h, --help show this help message and exit -t TARGET, --target TARGET define scan target -b, --background run port scanner in background with unix daemon, only support unix platform
Generate fuzz payload
Unicode Fuzz
from saker.fuzzer.code import Code
payload = Code.fuzzErrorUnicode(payload)
Fuzz SSI
from saker.fuzzers.ssi import SSI
payloads = [i for i in SSI.fuzz()]
Brute password or others
from saker.brute.dir import DirBrute
dirBrute = DirBrute("php", "index.php")
paths = dirBrute.weakfiles()
now support brute http basic auth, ftp, mysql, ssh, telnet, zipfile…
Call Third Party API
Crt.sh
from saker.api.crtsh import crtsh
crtsh("github.com")
DNSDumper
from saker.api.dnsdumper import DNSdumpster
DNSdumpster("github.com")
Github API
from saker.api.githubapi import GithubAPI
g = GithubAPI()
g.gatherByEmail("@github.com")
SQLMap API
from saker.api.sqlmap import SQLMap
options = {"url": "https://github.com"}
SQLMap().scan(options)
Handle HTML
import requests
from saker.handler.htmlHandler import HTMLHandler
r = requests.get("https://github.com")
h = HTMLHandler(r.text)
print(h.title)
The world’s leading software development platform · GitHub
print(h.subdomains("github.com"))
['enterprise.github.com', 'resources.github.com', 'developer.github.com', 'partner.github.com', 'desktop.github.com', 'api.github.com', 'help.github.com', 'customer-stories-feed.github.com', 'live-stream.github.com', 'services.github.com', 'lab.github.com', 'shop.github.com', 'education.github.com']
Special Server
from saker.servers.socket.dnsrebinding import RebindingServer
values = {
'result': ['8.8.8.8', '127.0.0.1'],
'index': 0
}
dnsServer = RebindingServer(values)
dnsServer.serve_forever()
Todo
APK analyze
Contributing
Contributions, issues and feature requests are welcome.
Feel free to check issues page if you want to contribute.
Disclaimer
This project is for educational purposes only. Do not test or attack any system with this tool unless you have explicit permission to do so.
Show your support
Please star this repository if this project helped you.
Issues
If you face any issue, you can create a new issue in the Issues Tab and I will be glad to help you out.
License
Copyright © 2019-2021 Lyle.
This project is GPLv3 licensed.
Leave a Reply